To remove an item from an ArrayList in Java based on its code, you can iterate over the ArrayList, check if the code matches, and then remove the item if it does. However, there are a few issues in your code that need to be addressed. Here’s an improved version:
javaimport java.util.ArrayList;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
ArrayList listBarang = new ArrayList<>();
listBarang.add("1101 TV");
listBarang.add("5531 Baju Baru");
listBarang.add("1425 Mesin Cuci");
Scanner input = new Scanner(System.in);
for (int i = 0; i < listBarang.size(); i++) {
System.out.println(listBarang.get(i));
}
System.out.println("Enter the code of the item you want to remove: ");
int code = Integer.parseInt(input.next());
boolean found = false;
for (int i = 0; i < listBarang.size(); i++) {
if (listBarang.get(i).startsWith(code + " ")) {
listBarang.remove(i);
found = true;
break;
}
}
if (!found) {
System.out.println("Code is invalid");
}
// Print updated list
System.out.println("Updated list:");
for (String item : listBarang) {
System.out.println(item);
}
}
}
In this code, we iterate over the ArrayList to find the item with the specified code. If the code matches, we remove the item and set found
to true. If no item with the specified code is found, we display “Code is invalid”. Finally, we print the updated list of items.
Note: This code assumes that each item in the ArrayList is in the format “
المزيد من المعلومات
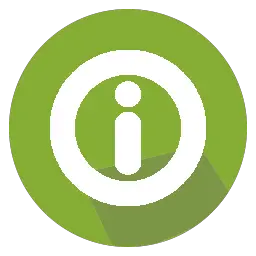
لتطبيق أكثر دقة في حذف العنصر من ArrayList بناءً على الرمز، يمكنك استخدام تعبيرات الاستدلال بواسطة Regex لتحديد العنصر بدقة. فيما يلي نسخة محسنة من الكود تستخدم هذه الطريقة:
javaimport java.util.ArrayList;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
ArrayList listBarang = new ArrayList<>();
listBarang.add("1101 TV");
listBarang.add("5531 Baju Baru");
listBarang.add("1425 Mesin Cuci");
Scanner input = new Scanner(System.in);
for (int i = 0; i < listBarang.size(); i++) {
System.out.println(listBarang.get(i));
}
System.out.println("Enter the code of the item you want to remove: ");
int code = Integer.parseInt(input.next());
boolean found = false;
for (int i = 0; i < listBarang.size(); i++) {
if (listBarang.get(i).matches("^" + code + " .*$")) {
listBarang.remove(i);
found = true;
break;
}
}
if (!found) {
System.out.println("Code is invalid");
}
// Print updated list
System.out.println("Updated list:");
for (String item : listBarang) {
System.out.println(item);
}
}
}
هذا الكود يستخدم matches("^" + code + " .*$")
للتحقق مما إذا كانت السلسلة تبدأ بالرقم المدخل ثم مسافة، وهذا يضمن تطابق الرمز بدقة دون تأثر بأي أرقام أخرى في السلسلة.